“PlayScript is an open source Adobe ActionScript compatible compiler and Flash compatible runtime that runs in the Mono .NET environment, targeting mobile devices through the Xamarin Studio MonoTouch and Mono for Android platforms” says the first sentence of its readme on GitHub. I have written this tutorial for those who want to quickly start playing with Playscript.
We will create simple iOS app In Xamarin Studio. This app will use native control (UIButton) and Actionscript 3 class which will supply button’s label. This tutorial targets developing with Monotouch. This means that you need corresponding license to compile the app on real device. It’s worth to note that all steps in this article is based on current versions of available binaries and source code (it’s 14 Apr 2013 today). And some of them may not be required in the future.
First of all, download compiled binaries (links in readme file) and source code from Playscript’s GitHub Page. Unpackage/unzip files and put them somewhere on your hard drive. We will assume that “ps_dir” is the full path to folder with compiled binaries and “ps_src_dir” – the same for source files.
Let’s add our new runtime to Xamarin Studio. To do this, go to Xamarin Studio > Preferences > .Net Runtimes, click Add button then navigate to your ps_dir and choose it. Then set is as default runtime. Completely quit Xamarin Studio and open it again.
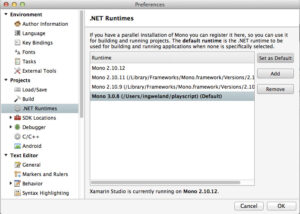
Now, we need to compile 2 assemblies from source: pscorlib_aot-monotouch.dll and Playscript.Dynamic_aot.dll. To do this, go to ps_src_dir/mcs/class/pscorlib_aot/ and open pscorlib_aot-net_4_0.sln solution. Next, right-click on solution and choose Add > Add Existing Project.
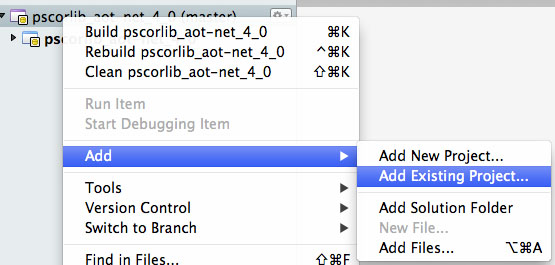
Navigate to ps_src_dir/mcs/class/pscorlib_aot/ directory and select pscorlib_aot-monotouch.csproj project. You will see following warning right after you add it.
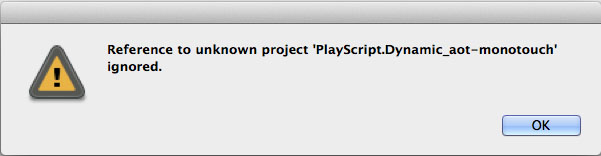
Thus, we need to add this missing project to our solution. It can be found in ps_src_dir/mcs/class/PlayScript.Dynamic_aot/ directory. After adding second project, you will notice that it has missing reference too – ICSharpCode.SharpZipLib.
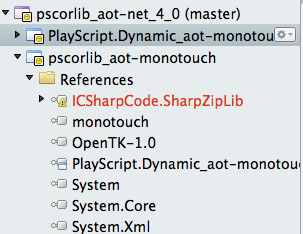
To fix this, right-click on References and then choose “Edit reference…”. We will take required dll from compiled binaries which we have downloaded. First of all, remove the ICSharpCode.SharpZipLib reference in “Selected references” list as it points to wrong location. Then go to “.Net Assembly” tab where you can navigate to the destination with required library. Hint: you can create links to your favorite folders there, with plus icon, this will save some time in the future. Find ICSharpCode.SharpZipLib.dll in ps_dir/lib/mono/gac/ICSharpCode.SharpZipLib/4-*.*/. Double click it or select and click “Add” button to add it to references list.
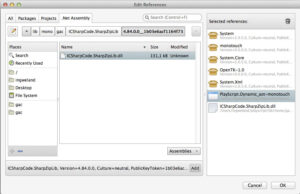
We are ready to compile. Clean solution by clicking on Build > Clean All.
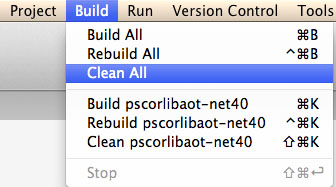
Do not build all projects at once, because many errors will popup from pscorlib_aot-net_4_0 project (technically, you can remove this project from solution or did any other required steps. But we will not cover this here as it’s not in the scope of the tutorial). Choose Release build. Right click on PlayScript.Dynamic_aot-monotouch project and build it. Then, do the same with pscorlib_aot-monotouch project.
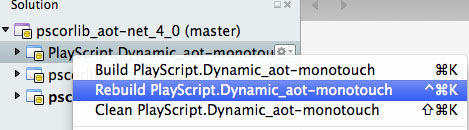
Now, we have our new DLLs in ps_src_dir/mcs/class/pscorlib_aot/bin/Release/. To keep things consistent, we will copy these libraries to our folder with other compiled binaries. Copy pscorlib_aot-monotouch.dll to ps_dir/lib/mono/gac/pscorlib_aot/1.*.*/ and PlayScript.Dynamic_aot.dll to ps_dir/lib/mono/gac/PlayScript.Dynamic_aot/1.*.*/.
We are ready to get hands dirty with real coding now. Create new solution and name it “HelloPlayscript“. Choose iOS > Universal > Single View Application as its type.
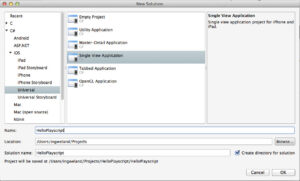
Right-click on References and select “Edit References…”. Find ICSharpCode.SharpZipLib.dll in “All” tab and add it. Then go to “.Net Assembly” tab, find and choose 2 assemblies which we have just compiled and copied.
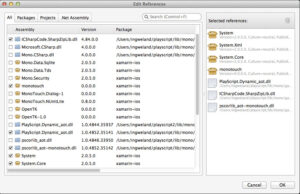
Add new text file to your project, name it ActionscriptWorld.as.
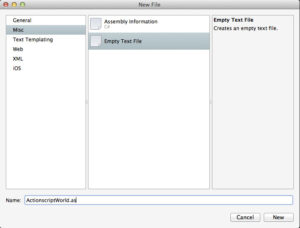
Important: you need to set Build action to Compile for Actionscript 3 files. To do it, right-click on the file and select Build Action > Compile.
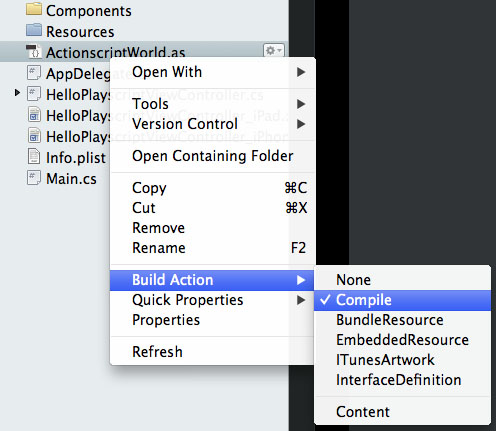
Paste following code into ActionscriptWorld.as (we need to explicitly specify radix in int.toString(), because current Playscript compiler does not default it to 10).
package { public class ActionscriptWorld { private var _labels:Vector.; private var _currentIndex:int = 0; public function ActionscriptWorld() { _labels = new Vector.(); _labels.push("Hello from Flash"); _labels.push("Yeah, I am Flash, indeed"); _labels.push("Why don't you believe in this?"); _labels.push("C'mon, stop clicking on me!"); _labels.push("Are you kidding?"); } public function getLabel():String { if(_currentIndex < _labels.length) { return _labels[_currentIndex++]; } else { return "You clicked me " + (++_currentIndex).toString(10) + " times!"; } } } }
Open HelloPlayscriptViewController.cs. Add “_root” namespace (this is current default namespace in Playscript. It’s likely to be changed in the future).
using _root;
Add new field which will reference the instance of our AS3 class.
ActionscriptWorld aw;
Because this is such a simple example, we will not bother with Interface Builder. We will do everything in code. Let’s instantiate our AS3 class, add Button to our view and create TouchUpInside delegate which will call the method in ActionscriptWorld and grab new label from it each time the user clicks the button. Note, that currently we do not have syntax highlighting etc support in Xamarin Studio. Also, references to Actionscript 3 classes and their members will be colored as errors. But compiler will not throw errors as long as the code itself does not have mistakes.
public override void ViewDidLoad() { base.ViewDidLoad(); aw = new ActionscriptWorld(); View.BackgroundColor = UIColor.White; RectangleF rect = new RectangleF(); rect.Width = View.Frame.Width; rect.Height = 88; rect.Y = (View.Frame.Height - rect.Height) / 2; UIButton myBtn = new UIButton(UIButtonType.RoundedRect); myBtn.Frame = rect; myBtn.SetTitle("Say \"Hello Flash!\"", UIControlState.Normal); myBtn.AutoresizingMask = UIViewAutoresizing.All; myBtn.TouchUpInside += delegate (object sender, EventArgs e) { myBtn.SetTitle(aw.getLabel(), UIControlState.Normal); }; View.AddSubview(myBtn); }
Run the app.
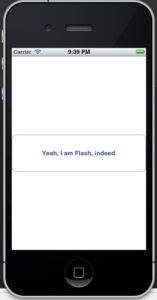
You have Actionscript 3 code compiled into your Monotouch app! You are ready to do some interesting and awesome things now!
Happy coding! 🙂
Great work, I’m very interested in PlayScript so thanks for the blog post.
Hello. Thanks for the article!
Could you give an example for android and show the connection android app layer with flash stage?
I believe that Android version should be pretty much similar (if we are talking about developing on MacOS). However, things has changed a bit since the time I wrote this tutorial. There are compiled binaries, intellisence and some new stuff in setting up demo projects. You should check Playscript’s GitHub page. I think I will write more tutorials in the future. But I want the things to settle down a bit, so tutorials will stay relevant for some time.
Thank you for answer. But i decided to run it on Windows, maybe later you can add tutorial about it?
Hi. Thanks for writing this up. When I try to run the app on Xamarin Studio I get the message that it is too large for the Starter Edition, even when just trying to deploy to the iPhone simulator. Is there a way to try this out without paying for a Xamarin license?
Hi!
Thanks for tutorial!
But I can’t build PlayScript.Dynamic_aot-monotouch and pscorlib_aot-monotouch.
I’ve following error:
https://dl.dropboxusercontent.com/u/573908/%D0%A1%D0%BD%D0%B8%D0%BC%D0%BE%D0%BA%20%D1%8D%D0%BA%D1%80%D0%B0%D0%BD%D0%B0%202013-07-06%20%D0%B2%2019.37.57.png
Have anyone any clue what can be wrong?
Already figured out. Problem was with folder name.
Hi Arthur,
Can you build this app with a Xamarin (free) starter license?
Thanks!
Duane.
As far as I know Starter edition will only limit the size of the app which can be deployed to the device. I think that you can have any size when building for simulator.
unfortunately the limit also holds for the simulator.
see http://xamarin.uservoice.com/forums/144858-xamarin-suggestions/suggestions/3693346-increase-the-starter-binary-size-to-more-than-32k
and the comments.
but you can get a trial license for a month to play with.
Arnoud
Hi!
I really like a trendyworkshop app from your portfolio. Is it possible to order app like that from you?
I sent you an e-mail